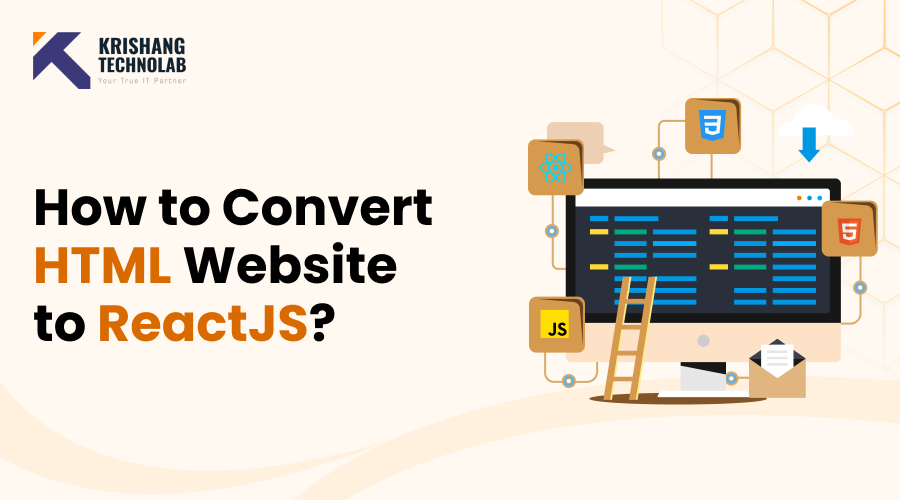
Quick overview: Convert HTML website to ReactJS can significantly enhance your site’s performance and maintainability. This process involves breaking down your static HTML into reusable React components, making the website more dynamic and interactive. By integrating React’s powerful features like state management, props, and hooks, you’ll be able to create a more flexible, efficient, and scalable application.
In today’s fast-paced digital world, having a static HTML website can limit your ability to create interactive, scalable, and high-performing applications. ReactJS, a powerful JavaScript library, has become a go-to solution for developers looking to enhance user experience and maintainability.
If you’re wondering how to convert HTML website into a dynamic ReactJS application, this guide will walk you through the entire convert HTML website to ReactJS process—step by step. Whether you’re a developer or a business owner looking to modernize your site, this guide will help you understand why and how to make the transition smoothly.
HTML vs. ReactJS: A Quick Comparison
Before diving into the migration process, let’s compare HTML and ReactJS to understand why this transition is beneficial.
Feature | HTML Website | ReactJS Application |
Interactivity | Mostly static | Highly dynamic |
Performance | Slower due to full-page reloads | Faster with Virtual DOM |
Code Reusability | Minimal | Component-based structure |
State Management | Requires manual updates | Handles state dynamically |
SEO Optimization | Good for simple sites | Requires SSR or pre-rendering for SEO |
When Should You Convert Your HTML Website to ReactJS?
- If your site requires frequent updates and maintenance.
- If you need a faster, more responsive UI.
- If your business demands a scalable and modular front-end.
Why ReactJS Is a Popular Choice for Modern Web Applications
ReactJS has gained immense popularity among developers and businesses due to its efficiency, flexibility, and scalability. Here are the key reasons why ReactJS stands out:
- Component-Based Architecture – React allows developers to build reusable UI components, making development faster and more maintainable.
- Virtual DOM for Performance – React’s Virtual DOM ensures efficient updates, improving rendering speed and overall performance.
- SEO-Friendly with SSR – While traditional React apps rely on client-side rendering, frameworks like Next.js enable server-side rendering (SSR) for better SEO.
- Rich Ecosystem and Community Support – A vast library of third-party tools and a strong developer community make React a preferred choice.
- Easy Integration with Backend APIs – React works seamlessly with RESTful APIs and GraphQL, making data handling more efficient.
- Cross-Platform Development – With React Native, developers can extend their web applications to mobile platforms using the same codebase.
Key Benefits of Converting an HTML Website to ReactJS
Upgrading from a static HTML website to a ReactJS-based application offers multiple advantages:
- Improved Performance – React’s Virtual DOM reduces unnecessary re-rendering, making your website faster.
- Better User Experience – Smooth animations, interactive UI, and real-time updates enhance user engagement.
- Scalability & Maintainability – The modular component structure makes it easier to add new features and maintain code.
- State Management for Dynamic Content – React’s useState and Context API allow dynamic data handling without page reloads.
- Enhanced Developer Productivity – Features like hot reloading speed up development and debugging.
- Future-Proof Technology – React is continuously evolving, ensuring long-term viability and support.
Read more about : The benefits of hiring ReactJS developers for web development
Key Considerations for Convert HTML Website to ReactJS
- Understand the Structure: Analyze static vs. dynamic content and clean up HTML.
- Componentization: Break the UI into reusable components and organize them hierarchically.
- State Management: Use React hooks or libraries like Redux for managing component or global state.
- Routing: Implement
react-router
for client-side navigation and dynamic routes. - JSX Syntax: Convert HTML to JSX, ensuring proper tag closures, attribute changes (e.g.,
class
toclassName
), and camel-casing. - CSS and Styling: Use CSS Modules, styled-components, or traditional CSS, and ensure responsiveness.
- Performance Optimization: Implement lazy loading, code splitting, and memoization to improve performance.
- Third-Party Libraries: Integrate existing JavaScript libraries, APIs, and any React-compatible versions.
- SEO Considerations: Use SSR (e.g., with Next.js) and implement dynamic meta tags for SEO.
- Testing and Debugging: Utilize Jest and React Testing Library for unit and integration testing, and React Developer Tools for debugging.
- PWA Features: Add service workers and caching for offline capabilities and push notifications.
- Deployment: Use platforms like Netlify or Vercel for smooth deployment after building the React app.
These considerations will help you transition from static HTML to a dynamic, maintainable ReactJS app.
Step-by-Step : Converting an HTML Website to ReactJS
Converting a static HTML website into a dynamic ReactJS application allows for better scalability, maintainability, and performance. Below is a detailed step-by-step guide on how to achieve this transformation.
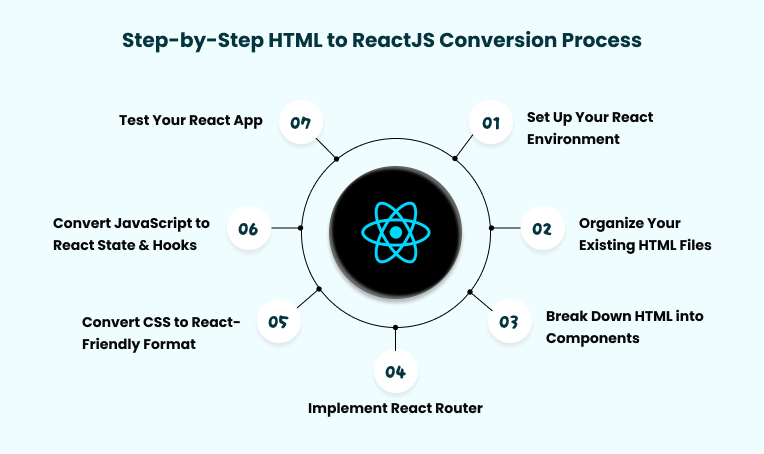
Step 1: Set Up Your React Environment
Before starting the conversion, ensure you have Node.js installed on your system. If not, download and install it from Node.js official website.
1.1 Install Node.js and npm
To check if Node.js and npm are installed, run: If they are not installed, download and install them.
node -v
npm -v
1.2 Create a React App
Use Create React App (CRA) to set up the project:
npx create-react-app my-react-app
cd my-react-app
Alternatively, if you prefer Vite for better performance:
npm create vite@latest my-react-app --template react
cd my-react-app
npm install
Step 2: Organize Your Existing HTML Files
2.1 Structure Your HTML Website
Your existing HTML site will typically consist of:
index.html
style.css
script.js
Additional assets like images, fonts, etc. Move these assets into the public
and src
folders of your React project.
2.2 Move Static Assets
- Place global assets (images, fonts, etc.) inside the
public
folder. - Move reusable CSS files inside
src/assets/css/
.
Step 3: Break Down HTML into Components
React works with reusable components, so you’ll need to break the HTML into small, manageable React components.
3.1 Identify Reusable Sections
For example, a simple HTML structure:
<header>
<h1>Welcome to My Website</h1>
</header>
<nav>
<ul>
<li><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
</ul>
</nav>
<main>
<h2>About Us</h2>
<p>This is an about page.</p>
</main>
<footer>
<p>© 2024 My Website</p>
</footer>
Can be divided into:
Header.js
Nav.js
Footer.js
Home.js
About.js
3.2 Create Components
Create a components
folder inside src/
and add files like Header.js
, Nav.js
, and Footer.js
.
Header Component (Header.js)
import React from 'react';
const Header = () => {
return (
<header>
<h1>Welcome to My Website</h1>
</header>
);
};
export default Header;
Navigation Component (Nav.js)
import React from 'react';
const Nav = () => {
return (
<nav>
<ul>
<li><a href="/">Home</a></li>
<li><a href="/about">About</a></li>
</ul>
</nav>
);
};
export default Nav;
Footer Component (Footer.js)
import React from 'react';
const Footer = () => {
return (
<footer>
<p>© 2024 My Website</p>
</footer>
);
};
export default Footer;
Main Content Components (Home.js & About.js)
import React from 'react';
const Home = () => {
return (
<main>
<h2>Home Page</h2>
<p>Welcome to the home page!</p>
</main>
);
};
export default Home;
import React from 'react';
const About = () => {
return (
<main>
<h2>About Us</h2>
<p>This is an about page.</p>
</main>
);
};
export default About;
Step 4: Implement React Router
To navigate between pages, install React Router:
npm install react-router-dom
Modify App.js
to use routing:
import React from 'react';
import { BrowserRouter as Router, Routes, Route } from 'react-router-dom';
import Header from './components/Header';
import Nav from './components/Nav';
import Footer from './components/Footer';
import Home from './pages/Home';
import About from './pages/About';
function App() {
return (
<Router>
<Header />
<Nav />
<Routes>
<Route path="/" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
<Footer />
</Router>
);
}
export default App;
Step 5: Convert CSS to React-Friendly Format
If your CSS is global, you can import it inside index.css
or App.css
:
import './assets/css/style.css';
For module-based styling, rename CSS files to .module.css
and use them like this:
import styles from './Header.module.css';
const Header = () => {
return (
<header className={styles.header}>
<h1>Welcome</h1>
</header>
);
};
Step 6: Convert JavaScript to React State & Hooks
If your HTML site has a JavaScript file (script.js
) that manages interactivity, convert it into React state and hooks.
Example: Handling a Button Click
Before (HTML + JS):
<button id="clickMe">Click Me</button>
<script>
document.getElementById("clickMe").addEventListener("click", function () {
alert("Button clicked!");
});
</script>
After (React + Hooks):
import React, { useState } from 'react';
const ClickButton = () => {
const [message, setMessage] = useState('');
const handleClick = () => {
setMessage('Button clicked!');
};
return (
<div>
<button onClick={handleClick}>Click Me</button>
{message && <p>{message}</p>}
</div>
);
};
export default ClickButton;
Step 7: Test Your React App
To run your new React website:
npm start
Your site should now be running at http://localhost:3000/
.
Step 8: Deploy the React App
Once everything is working fine, deploy your React app.
8.1 Build the Production Version
npm run build
8.2 Deploy to GitHub Pages
- Install
gh-pages
npm install gh-pages --save-dev
- Update
package.json
"homepage": "https://yourusername.github.io/your-repo-name",
"scripts": {
"predeploy": "npm run build",
"deploy": "gh-pages -d build"
}
- Deploy:
npm run deploy
Alternatively, you can deploy via Vercel, Netlify, or Firebase.
Conclusion
Converting an HTML website to ReactJS opens up a world of possibilities for enhancing user experience, scalability, and maintainability. By breaking down your static pages into reusable components, integrating state management with hooks, and utilizing React Router for seamless navigation, you’re laying the groundwork for a more dynamic and responsive web application.
With React’s powerful features, like component-based architecture and efficient rendering, your site will be better equipped to handle future updates, feature additions, and performance optimization. Once deployed, your new ReactJS website will be ready to deliver a modern, engaging experience for your users.
This guide has provided a clear, step-by-step approach to help you make the transition. Now it’s time to test your application, deploy it, and start enjoying the benefits of a React-powered website.
Why Krishang Technolab is Your Ideal Partner for Convert HTML Website to ReactJS?
Krishang Technolab is a leading ReactJS development company, recognized for delivering cutting-edge, scalable, and user-friendly solutions. Our team of experts ReactJS developers excels at converting HTML websites into ReactJS with minimal disruption, ensuring improved performance, enhanced interactivity, and superior user experiences.
We cater to businesses of all sizes, from startups to established enterprises, offering tailor-made solutions designed to meet your unique business objectives. By leveraging the power of ReactJS, we help businesses build future-proof websites that are flexible, responsive, and optimized for growth.
Why Choose Krishang Technolab for HTML Website to Reactjs?
- Expertise in ReactJS: Krishang Technolab offers deep knowledge and hands-on experience in ReactJS development, ensuring a smooth transition for your HTML website.
- Seamless Integration: We make sure your HTML website converts flawlessly into ReactJS without compromising design or functionality.
- Customized Solutions: Our team tailors ReactJS integration to your specific business needs, enhancing both user experience and performance.
- Faster Development: With our efficient approach, we speed up the conversion process, reducing the time-to-market for your updated website.
- Scalability: Post-conversion, your ReactJS website will be scalable and future-proof, ready to grow with your business.
- Cost-Effective: Our Reactjs development Services provide high value with affordable pricing, giving you the best of both worlds.
- Ongoing Support: We offer continuous support even after the conversion, ensuring your website runs smoothly.